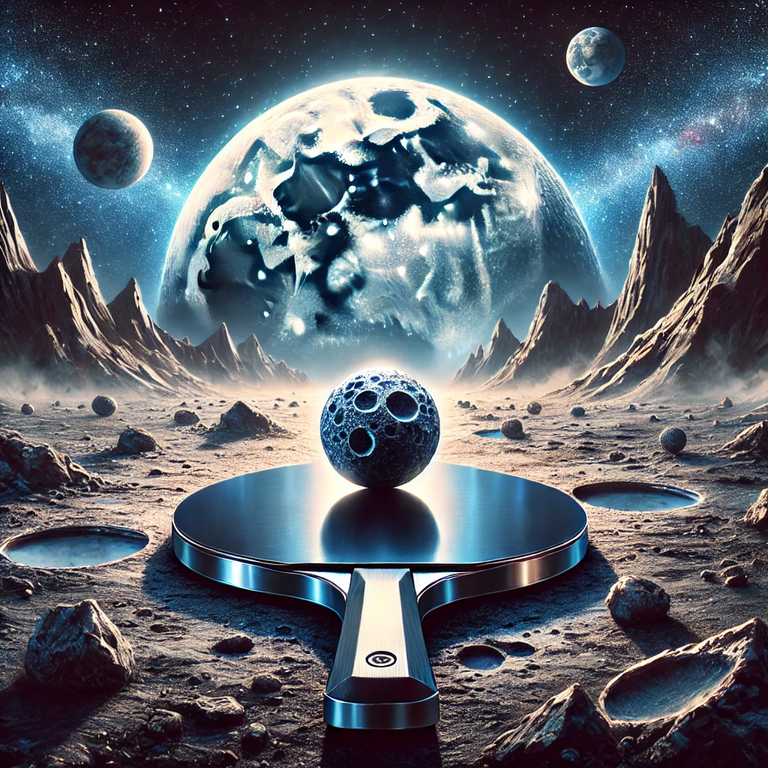
I appreciate the help @oldsoulnewb I definitely needed more guidance with the Markdown Language limits.
That said, I spent the past week working on this Pong Game. I have to figure out still how to auto-reset the "asteroid" and keep a score. I might work on the menu next. We'll go for a dry run and see if I can embed it on my Geocities website. If I can I'll edit this post and in place of what I'm writing now I'll put the link.
Feel free to use it as you wish. If you edit this to anything cooler please tag me in it, so we can nerd out together.
lunar_pong.py
import pygame as moonlight
import sys as celestial
# Initialize moonlight
moonlight.init()
# Constants
MOON_SURFACE_WIDTH = 800
MOON_SURFACE_HEIGHT = 600
LUNAR_DARK = (0, 0, 0) # Dark Moon background
CRATER_COLOR = (255, 255, 255) # White paddles
ASTEROID_COLOR = (255, 255, 255) # White ball
CRATER_WIDTH = 10
CRATER_HEIGHT = 100
ASTEROID_SIZE = 10
CRATER_SPEED = 6
ASTEROID_SPEED_X = 4
ASTEROID_SPEED_Y = 4
# Create the moon surface
surface = moonlight.display.set_mode((MOON_SURFACE_WIDTH, MOON_SURFACE_HEIGHT))
moonlight.display.set_caption('Lunar Pong')
# Craters
crater1 = moonlight.Rect(30, (MOON_SURFACE_HEIGHT // 2) - (CRATER_HEIGHT // 2), CRATER_WIDTH, CRATER_HEIGHT)
crater2 = moonlight.Rect(MOON_SURFACE_WIDTH - 30 - CRATER_WIDTH, (MOON_SURFACE_HEIGHT // 2) - (CRATER_HEIGHT // 2), CRATER_WIDTH, CRATER_HEIGHT)
# Asteroid
asteroid = moonlight.Rect((MOON_SURFACE_WIDTH // 2) - (ASTEROID_SIZE // 2), (MOON_SURFACE_HEIGHT // 2) - (ASTEROID_SIZE // 2), ASTEROID_SIZE, ASTEROID_SIZE)
# Movement variables
asteroid_speed_x = ASTEROID_SPEED_X
asteroid_speed_y = ASTEROID_SPEED_Y
crater1_speed = 0
crater2_speed = 0
# Main lunar loop
orbiting = True
while orbiting:
for event in moonlight.event.get():
if event.type == moonlight.QUIT:
orbiting = False
if event.type == moonlight.KEYDOWN:
if event.key == moonlight.K_w:
crater1_speed = -CRATER_SPEED
if event.key == moonlight.K_s:
crater1_speed = CRATER_SPEED
if event.key == moonlight.K_UP:
crater2_speed = -CRATER_SPEED
if event.key == moonlight.K_DOWN:
crater2_speed = CRATER_SPEED
if event.type == moonlight.KEYUP:
if event.key == moonlight.K_w or event.key == moonlight.K_s:
crater1_speed = 0
if event.key == moonlight.K_UP or event.key == moonlight.K_DOWN:
crater2_speed = 0
# Move craters
crater1.y += crater1_speed
crater2.y += crater2_speed
# Keep craters within the moon surface
if crater1.top < 0:
crater1.top = 0
if crater1.bottom > MOON_SURFACE_HEIGHT:
crater1.bottom = MOON_SURFACE_HEIGHT
if crater2.top < 0:
crater2.top = 0
if crater2.bottom > MOON_SURFACE_HEIGHT:
crater2.bottom = MOON_SURFACE_HEIGHT
# Move asteroid
asteroid.x += asteroid_speed_x
asteroid.y += asteroid_speed_y
# Asteroid collision with top/bottom
if asteroid.top <= 0 or asteroid.bottom >= MOON_SURFACE_HEIGHT:
asteroid_speed_y *= -1
# Asteroid collision with craters
if asteroid.colliderect(crater1) or asteroid.colliderect(crater2):
asteroid_speed_x *= -1
# Fill the background
surface.fill(LUNAR_DARK)
# Draw craters and asteroid
moonlight.draw.rect(surface, CRATER_COLOR, crater1)
moonlight.draw.rect(surface, CRATER_COLOR, crater2)
moonlight.draw.ellipse(surface, ASTEROID_COLOR, asteroid)
# Update the moon surface
moonlight.display.flip()
# Quit moonlight
moonlight.quit()
celestial.exit()