With this post, I'll help you understand how to generate a curation report.
Prerequisites
- Node.js basic knowledge
- Node.js 16 installed on your system.
Step 1: Install dependencies
- install following dependencies (with
npm i
)
dotenv
axios
@blurtfoundation/blurtjs
moment
fs
Step 2: Load dependencies
- Create a file
gen_report.js
const dotenv = require("dotenv");
dotenv.config();
var axios = require("axios");
var blurt = require("@blurtfoundation/blurtjs");
var moment = require("moment");
var fs = require("fs");
Step 3: Create .env
file
- Create
.env
file
- Add
BLURT_RPC
value.
- It could be any of the RPC node value
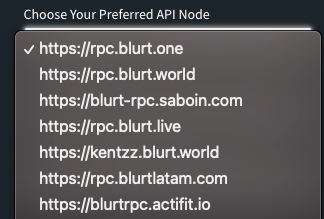
Step 4: Set up blurt RPC & create yesterday date.
blurt.api.setOptions({ url: process.env.BLURT_RPC, useAppbaseApi: true });
const now = moment.now();
const newNow = moment(now).subtract(1, "days");
const newNowFormatted = moment(newNow).utcOffset("+05:30").format();
const newNowFormattedDate = newNowFormatted.split("T")[0];
Step 5: Set up curation report header & footer.
const FOOTER = `your curation report footer goes here`;
const HEADER = `your curation report header goes here`;
Step 6: A function to load upvote data
- Copy & paste following function.
- Make sure to change
sagarkothari88
from following function to your name.
- It will avoid adding your upvotes of your own content.
function getUpvoteData(ofUser, limit) {
return new Promise(function (resolve, reject) {
var data = {
id: "1",
jsonrpc: "2.0",
method: "call",
params: ["condenser_api", "get_account_history", [ofUser, -1, limit]],
};
var config = {
method: "post",
url: process.env.BLURT_RPC,
data: data,
};
axios(config)
.then(function (response) {
const result = response.data.result;
var items = [];
for (let item in result) {
const operation = result[item][1]["op"];
const operationType = operation[0];
const operationData = operation[1];
if (
operationType !== undefined &&
operationType === "vote" &&
operationData["author"] !== "sagarkothari88"
) {
items.push({
author: operationData.author,
permlink: operationData.permlink,
weight: operationData.weight,
datetime: result[item][1].timestamp,
});
}
}
resolve(items);
})
.catch(function (error) {
console.log(error);
reject(error);
});
});
}
Step 7: Load yesterday's upvotes
- Copy paste following function
- Make sure to change
sagarkothari88
to your name.
- Make sure to change timezone to your timezone.
async function getUpvotedData() {
var result = await getUpvoteData("sagarkothari88", 4000);
var items = [];
for (let item in result) {
const timeStamp = result[item].datetime;
const dateTime = moment(timeStamp, moment.ISO_8601)
.utcOffset("+05:30")
.format()
.split("T")[0];
if (dateTime === newNowFormattedDate) {
const entry = `| @${result[item].author} | https://blurt.blog/@${result[item].author}/${result[item].permlink} |`;
items.push(entry);
}
}
return items;
}
Step 8: A function to print curation report to file.
- Copy paste following function
- Make sure to change timezone to your timezone.
async function printReport() {
console.log(
`Started at ${moment(moment.now()).utcOffset("+05:30").format()}`
);
let text = HEADER;
const data = await getUpvotedData();
text = `${text}\n${data.join("\n")}`;
text = `${text}\n${FOOTER}`;
console.log(`Ended at ${moment(moment.now()).utcOffset("+05:30").format()}`);
fs.writeFileSync("./blurt_report.txt", text);
}
Step 9: Generate report by executing the above function.
printReport();
Step 10: Copy paste curation report
- Locate
blurt_report.txt
- Copy paste into your post