In JavaScript and TypeScript, a Promise must resolve or reject before the subsequent line of code can be performed; hence, await is used to wait for that action.
When the async keyword is applied before a function, an async function with a Promise return value is produced.
Use the await keyword before the Promise to stop a function within an async function from running until the Promise resolves or rejects.
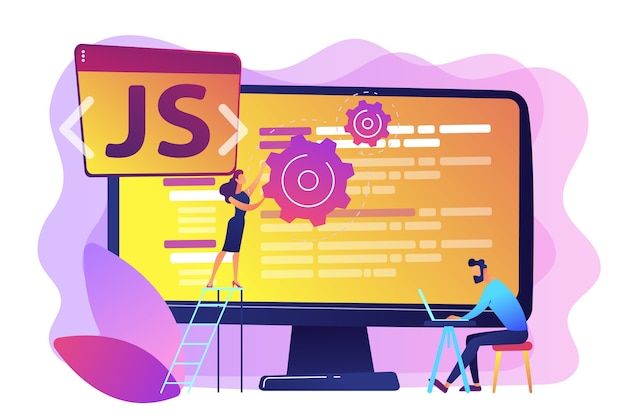
src
When dealing with complicated asynchronous activities, using await can make the code more understandable and simpler to reason about. In order to handle Promise resolution and rejection without await, we would need to use.then() and.catch(), which can result in "callback hell" and difficult-to-read code.
An illustration of how await might make the code more is shown in the following example:
// Without await
fetch("https://example.com/data")
.then((response) => {
return response.json();
})
.then((data) => {
console.log(data);
})
.catch((error) => {
console.error(error);
});
// With await
async function fetchData() {
try {
const response = await fetch("https://example.com/data");
const data = await response.json();
console.log(data);
} catch (error) {
console.error(error);
}
}
fetchData();
.then() and.catch() are used in the first example to handle the Promise resolution and rejection, respectively. Using await to halt the execution until the Promise resolves or rejects in the second example might help make the code more legible and understandable.
Now There can be a question from this Line:-
"Within an async function, we can use the await keyword before a Promise to pause the execution of the function until the Promise resolves or rejects"
Here's an example of an async function until a Promise resolve:
async function exampleFunction() {
console.log("Before Promise");
const result = await new Promise((resolve) => {
setTimeout(() => {
console.log("Inside Promise");
resolve("Promise Resolved");
}, 2000);
});
console.log(`After Promise: ${result}`);
}
exampleFunction();
We define an async function in this example named exampleFunction. Before constructing a new Promise that resolves after a 2-second delay, we first report a message to the console inside the function.
To halt the function's execution until the Promise resolves, we use the await keyword before the Promise. The result variable receives the value that was used to resolve the promise when it did so.
The result variable is added to the console message. The messages are logged, as can be seen:
Before Promise
Inside Promise
After Promise: Promise Resolved
Congratulations, your post has been curated by @r2cornell, a curating account for @R2cornell's Discord Community.