Overview of React Flow
For creating interactive node-based interfaces with React, there is a package called React Flow. Visual diagrams, flowcharts, mind maps, and other interactive visuals that the user may change are simple to make using React Flow. On top of the well-known React framework, React Flow offers a straightforward and adaptable API for generating and modifying nodes and edges.
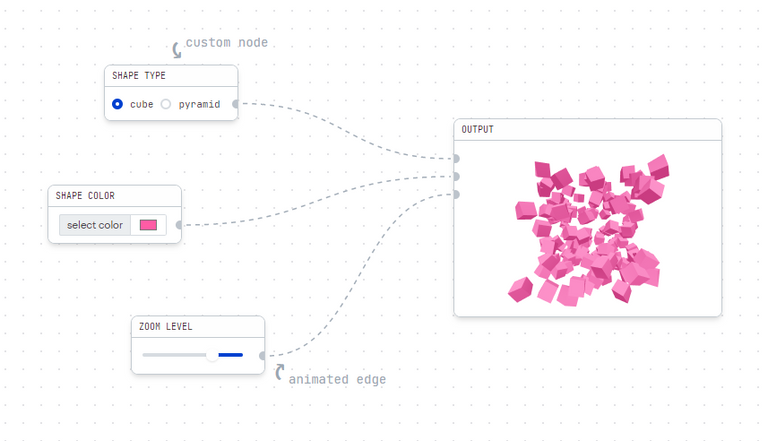
src
Getting Going
You'll need to have a fundamental grasp of React and JavaScript in order to get started with React Flow.
npm install reactflow
or
yarn add reactflow
Once installed, you can import React Flow and start building your application:
import ReactFlow from 'reactflow';
import "reactflow/dist/style.css";
function MyFlow() {
return <ReactFlow />;
}
This will render an empty flow canvas on the page.
Adding Nodes and Edges To add nodes to your flow, you can use the nodes and edges prop:
import ReactFlow from 'reactflow';
import "reactflow/dist/style.css";
const nodes= [
{ id: '1', type: 'input', data: { label: 'first Node' }, position: { x: 0, y: 0 } },
{ id: '2', type: 'output', data: { label: 'Second Node' }, position: { x: 100, y: 100 } },
{ id: '3', type: 'default', data: { label: 'Third Node' }, position: { x: 200, y: 200 } }
];
function MyFlow() {
return <ReactFlow nodes={nodes} />;
}
Three nodes—an input node, an output node, and a default node—will be displayed on the flow canvas as a result. The position attribute establishes the node's initial location on the canvas.
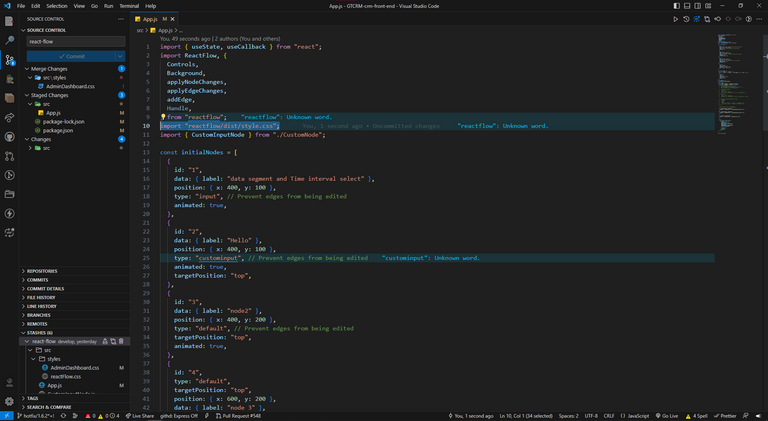
ScreenShot
To connect nodes with edges, you can use the edges props:
import ReactFlow from 'reactflow';
import "reactflow/dist/style.css";
const nodes= [
{ id: '1', type: 'input', data: { label: 'first Node' }, position: { x: 0, y: 0 } },
{ id: '2', type: 'output', data: { label: 'second Node' }, position: { x: 100, y: 100 } },
{ id: '3', type: 'default', data: { label: 'Third Node' }, position: { x: 200, y: 200 } }
];
const edges = [
{ id: 'e1-2', source: '1', target: '2' },
{ id: 'e2-3', source: '2', target: '3' }
];
function MyFlow() {
return <ReactFlow nodes={nodes} edges={edges} />;
}
Conclusion
A potent library for using React to build interactive node-based interfaces is React Flow. Its straightforward and adaptable API makes it simple to make intricate flowcharts and other interactive images. Utilize it in your upcoming React project!
Congratulations, your post has been curated by @r2cornell, a curating account for @R2cornell's Discord Community.